Learning React(JS) the hard way Part 2
Hey friend, this is the second part of my small series about me getting introduced to JS.
- If you're here by accident and actually wanted to read the whole story click here.
- If you're just interested in my first impression of React and you don't actually care about the background, keep on reading.
- These are definitely not the druids you are looking for.
We are definitely not close
In late 2018 I started working as a python backend developer for a ML driven platform which basically matches future employers, so called job candidates with jobs within hours (sometimes a few days).
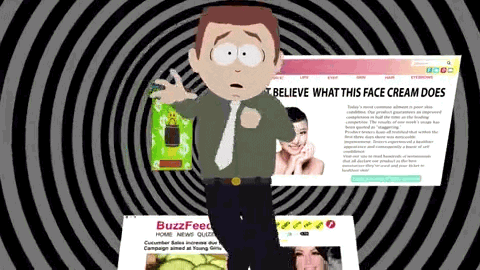
enough ads!
During december 2018 my boss introduced me to the idea of being a full stack developer. As far as I remember I said during my interview for exact this job position
Please don't ask me to do frontend tasks, I hate the accuracy you need to vertical align two divs
Now I hear people saying "Just use the damn flex-box
, idiot". But let's keep to the fact that you have thousands of
different attributes which can define the exact same state. And it's just too hard for me to decide whether to use low-level
attributes like left
instead of a margin-left
, or chose order
because the fancy flex
gives me purrrrfect alignment.
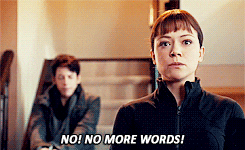
Anyway I didn't refuse the offer and started learning JavaScript – as mentioned in my last post.
Which turns out to be the greatest idea of my whole life?
Don't think so.
Yes, I use JS in multiple projects, since I learned it. But isn't that just common sense? Why to forget something because you don't like it? Is it the fear of incompatibility with your current tech stack?
For me it was just inconvenient to learn those "silly" languages, as C++, C, R, MATLAB and SAS. In my perspective I feel like I've traveled a lot through languages and was happily surprised when I dug into Python by writing small helpful scripts. To be hones... it's easy. A few rules to follow and the sky is the limit 🚀.
But today I'm here to tell you something about my experience with JavaScript and especially my dear friend ReactJS.
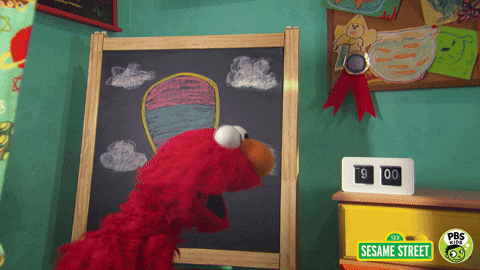
After reading through several topics of "The Modern JavaScript Tutorial", scripting a few things aaaaaaaaand the beautiful support through the fantastic Tutorial: Intro to React I felt wise enough to start writing my first small page using react and nextJS for SSR support. I also needed to set up an express server to achieve SSR support. The schema is a bit different to regular React because of nextjs, but I guess you'll get the gist, since I got it. ;)
So I ended up with a directory like that
with my index.js
:
import React from 'react'
export class App extends React.Component {
render() {
return (
<div>
<h1>Hello World!</h1>
</div>
)
}
}
export default App
and server.js
const express = require('express')
const next = require('next')
const port = parseInt(process.env.PORT, 10) || 3000
const dev = process.env.NODE_ENV !== 'production'
const app = next({ dev })
const handle = app.getRequestHandler()
app.prepare().then(() => {
const server = express()
server.get('*', (req, res) => {
return handle(req, res)
})
server.listen(port, err => {
if (err) throw err
console.log(`> Ready on http://localhost:${port}`)
})
})
So I started with my first version of a "Component", because components are React or is React components? (/•-•)/:
1st version:
import React from 'react'
export class MyComponent extends React.Component {
render() {
return (
<div>
<h1>Hello World!</h1>
</div>
)
}
}
export default MyComponent
2nd version:
import React from 'react'
export const MyComponent = () => {
return (
<div>
<h1>Hello World!</h1>
</div>
)
}
export default MyComponent
3rd version:
import React from 'react'
export const MyComponent = () => (
<div>
<h1>Hello World!</h1>
</div>
)
export default MyComponent
As you can see the evolution of this component is from a
"React Class Component"
to a "React Functional Component".
Maybe you get my point already, but let's say we add for example some functionality to our component. Let's pass a variable
"title
" through to display within our h1
-tag.
For convenience I continue with version 1 and 2, because 3 is obviously just a shorter version of 2
1st version:
import React from 'react'
export class MyComponent extends React.Component {
render() {
return (
<div>
<h1>{this.props.title}</h1>
</div>
)
}
}
export default MyComponent
2nd version:
import React from 'react'
export const MyComponent = ({title}) => {
return (
<div>
<h1>{title}</h1>
</div>
)
}
export default MyComponent
The usage of our components is equal, we can use both within pages/index.js
:
import React from 'react'
+import MyComponent from '../src/components/MyComponent'
export class App extends React.Component {
render() {
return (
- <div>
+ <MyComponent title={'Hello World!'}/>
- </div>
)
}
}
export default App
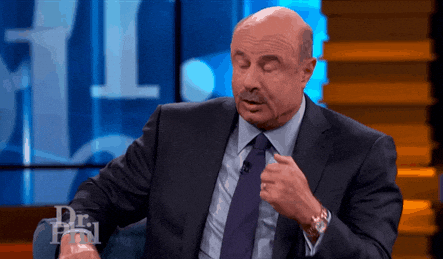
Incredible website, I know!
But as you can imagine, this is just one way to do it. There're several other ways to reach one goal. And different options can end in different scenarios. So be careful by choosing the wrong component type!
Here's a tip from my side, which might be wrong, but it works pretty good for me. I always start with class components
JavaScript and especially ReactJS as a framework update their standards so frequently, that I don't feel super confident about big JavaScript projects. But why not trying to keep your code base up to date! In my opinion it's not a goal to refactor your whole project once per year, just because there's a new fancy way of doing it. Why should we change a running system?
In that moment most of the people think about the possible options how to use this and how awesome it will be in the future. All I can think about is:
Dude! That wasn't even 10 minutes and you can basically increase your productivity by quitting to use basic HTML and use React even though you're building a static page.
Reusable Com... Com...
Reusable Components
Sorry again friend, I need to postpone the topic about packages and libraries for Post #3 Learning React(JS) the hard way Part 3. If you like reading so far and want to give me nice feedback hit me per Mail or try to contact me in other known ways.
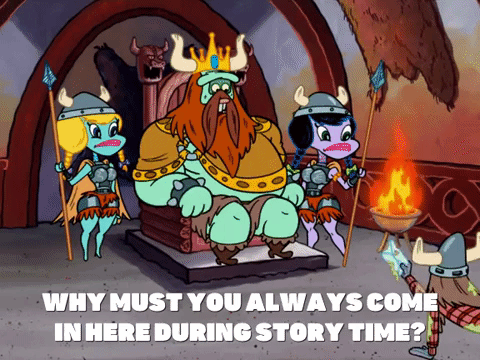
Enjoy your weekend
Phil
Credits:
Title Image: Gareth Harrison